Algorithm Basics: A Beginner’s Guide to Sorting and Searching
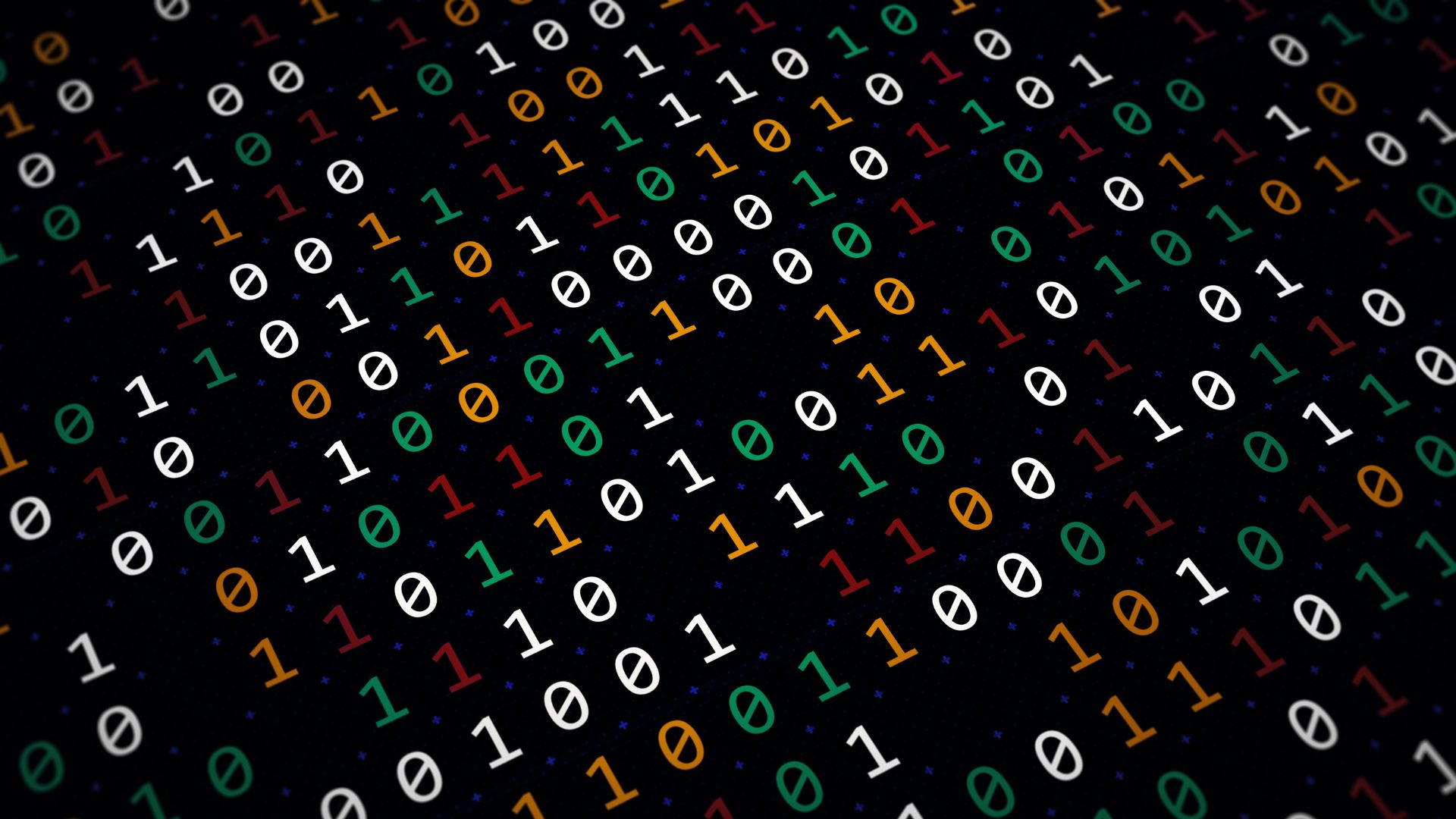
Algorithms play a crucial role in computing, enabling computers to efficiently process data and solve problems. Among the most fundamental are sorting and searching algorithms, which help organize and locate information quickly.
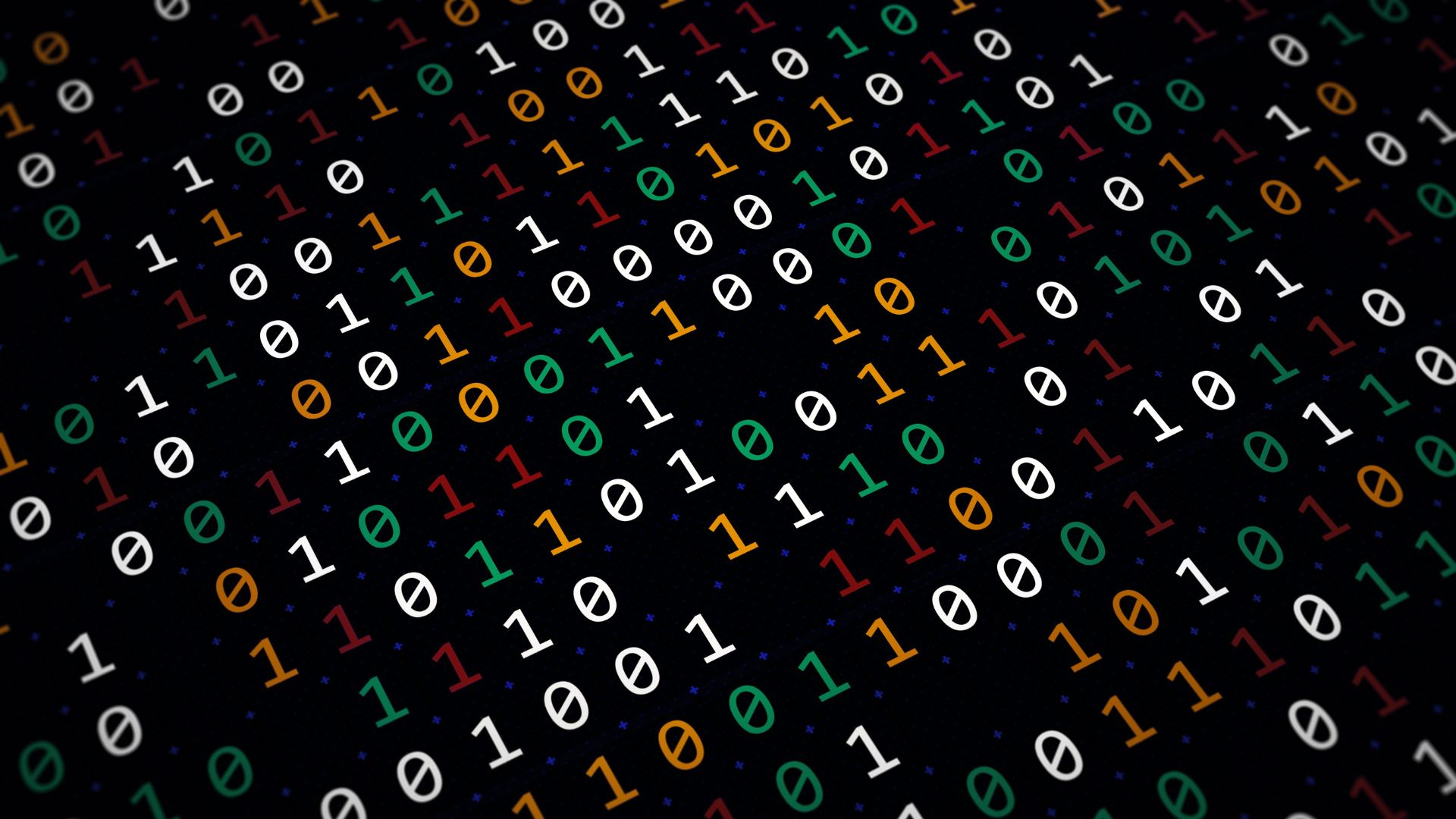
Understanding Sorting Algorithms
Sorting arranges data in a specific order, typically ascending or descending. Some of the most commonly used sorting algorithms include:
- Bubble Sort: A simple algorithm that repeatedly swaps adjacent elements until the list is sorted. Though easy to understand, it is inefficient for large datasets.
- Merge Sort: A divide-and-conquer algorithm that splits the list into smaller parts, sorts them, and then merges them back together. It has a time complexity of O(n log n).
- Quick Sort: Another divide-and-conquer approach that selects a pivot element and partitions the list around it. It often outperforms merge sort in practice.
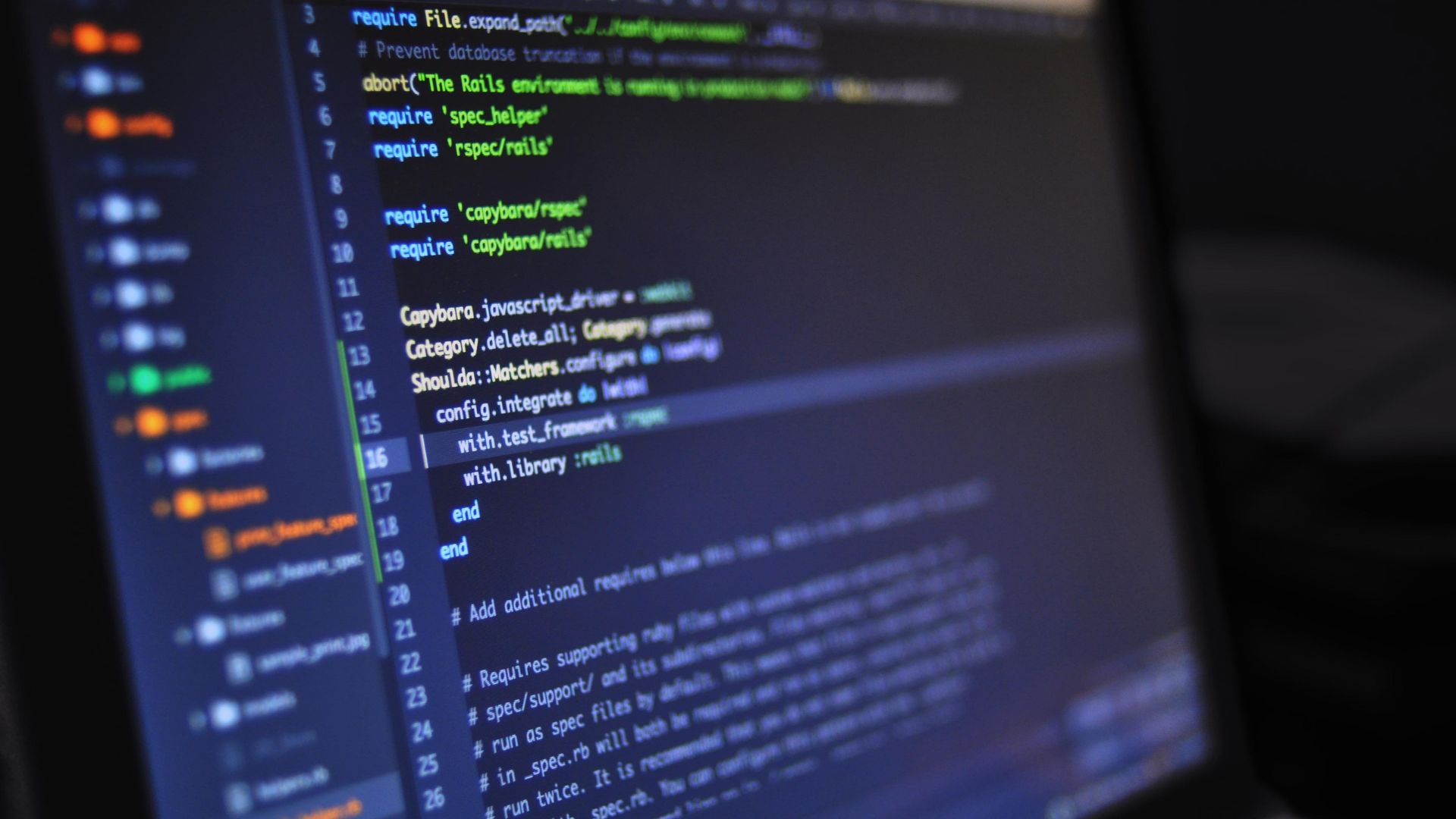
Understanding Searching Algorithms
Searching algorithms help find specific elements in a dataset. The two main types are:
- Linear Search: A straightforward approach that checks each element one by one until a match is found. It has a worst-case time complexity of O(n).
- Binary Search: A much faster method that repeatedly divides a sorted list in half until the target element is found. It runs in O(log n) time, making it significantly more efficient than linear search for large datasets.
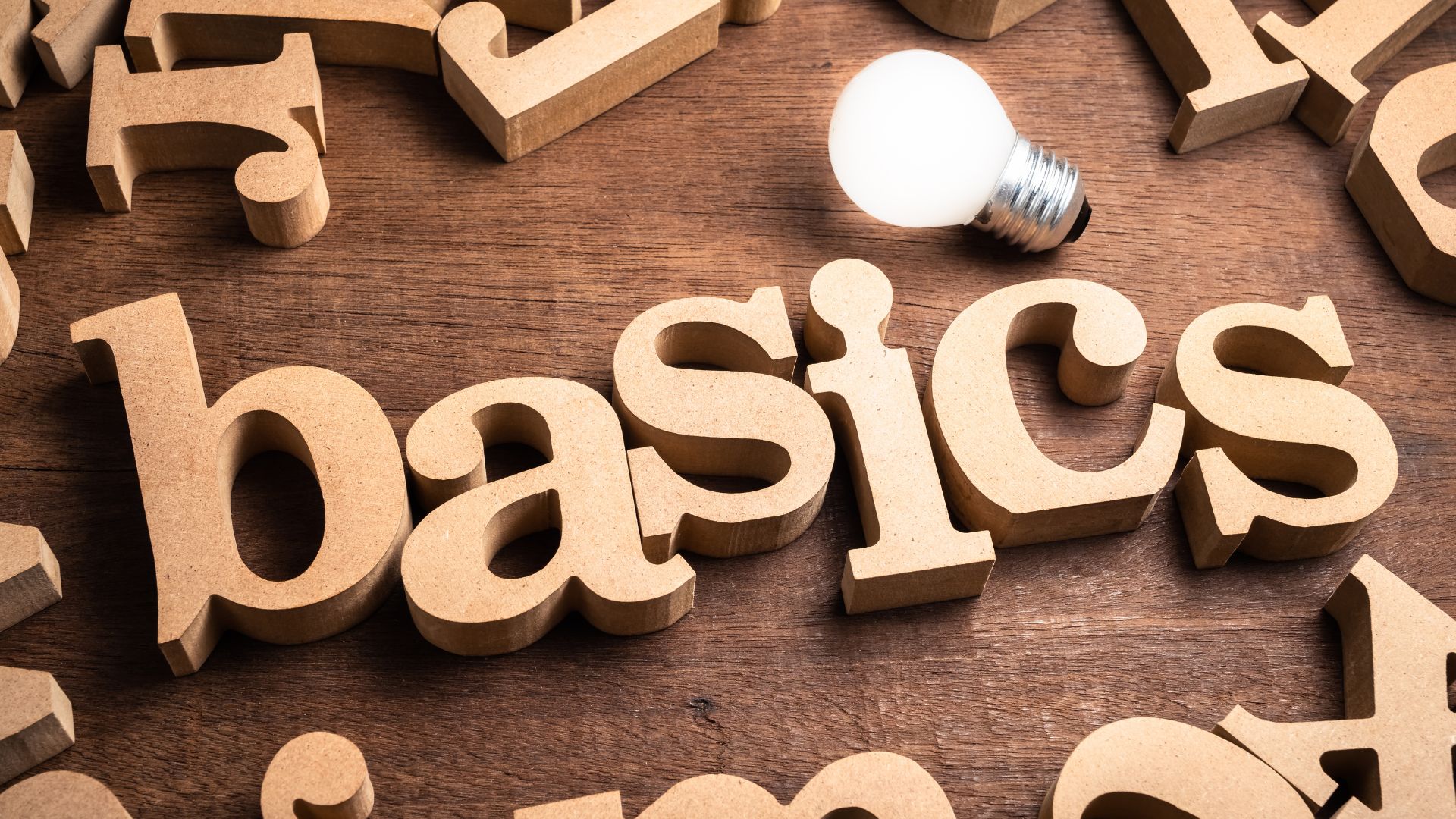
Why Sorting and Searching Matter
Sorting and searching algorithms are used in everyday applications, from database indexing to recommendation systems. Mastering these basics is essential for anyone learning computer science or working with data structures.
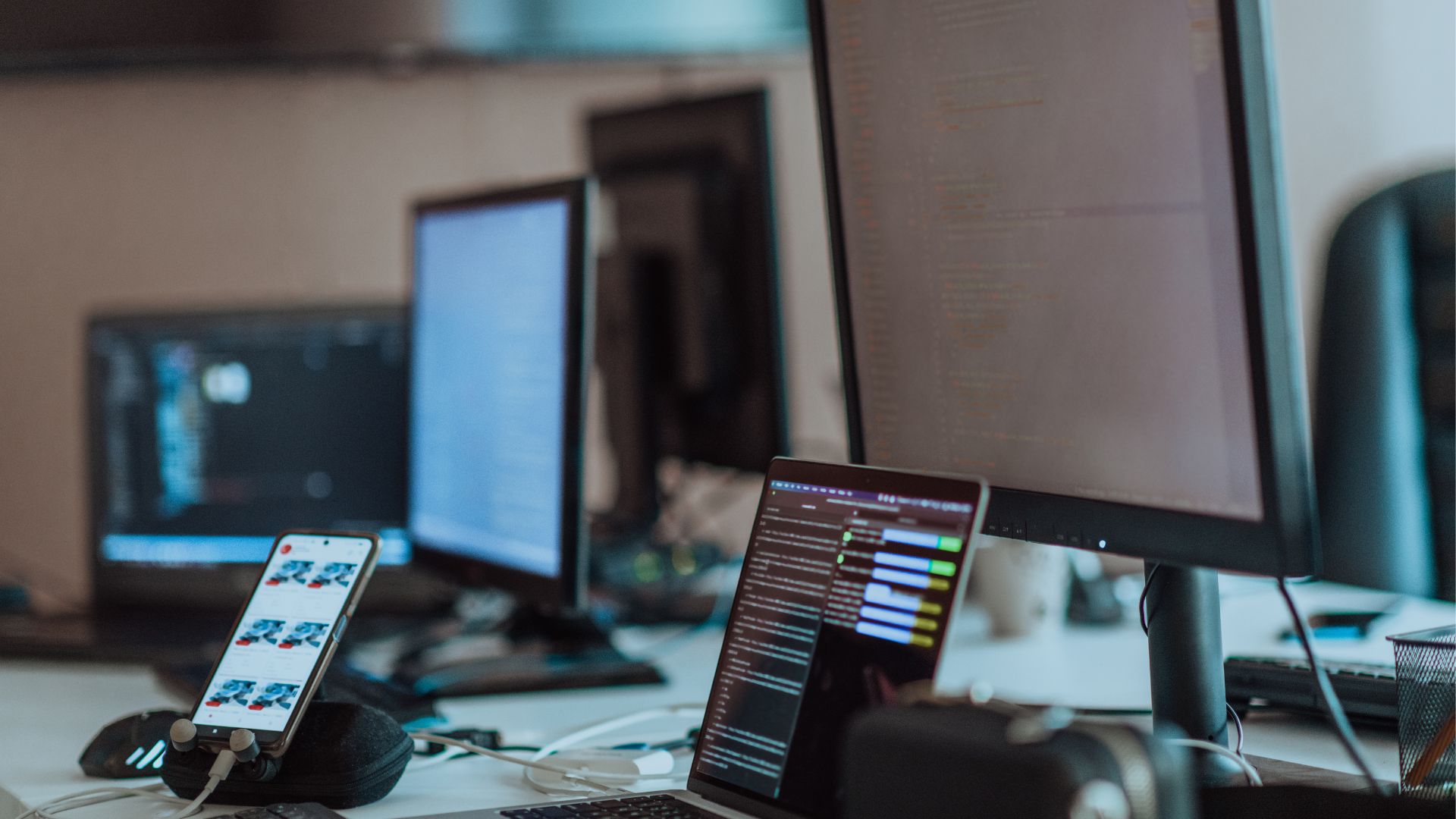